Sometimes even an easiest task can be daunting especially when you have just started learning a programming language. This tutorial is for python programmers who have started learning the language.
What is an odd number?
Mathematically speaking, if a number is not fully divisible by 2 then it is an odd number. To check whether or not a given number is an odd number then we just need to look up to its modulus. In Python, to find modulus (remainder) of a division we use modulo operator (which is “%”).
You can read more in detail in the Wikipedia’s article.
How to use modulo operator?
Instead of using normal division operator “/”, we’ll use modulo operator “%” for modulo operation. Writing following line in Python shell will give us modulus (remainder) of the division of 9 / 2
:
9 % 2
Output is as following:
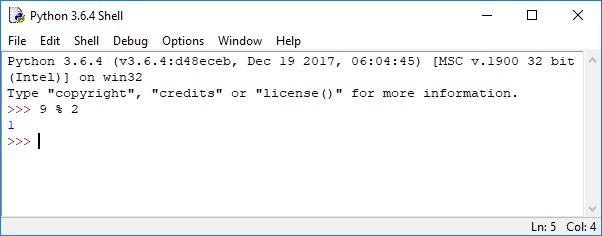
Finding all odd numbers between 0 and 100
In Python, if a number is completely divisible by 2 then the modulus will be 0 for an even number. However, if modulus is 1 then that would be an odd number. With that knowledge in our hand, we can write a For-Loop
to find odd numbers between 0 and let’s say 100.
for number in range(100): if number % 2 > 0: print("{0} is an odd number".format(number))
Find all odd numbers up to user’s input
Instead of fixed number for our For-Loop
in above code, we can ask user to give us a number to calculate odd numbers up to that number:
max_number = int(input("Enter a number: ")) for number in range(max_number): if number % 2 > 0: print("{0} is an odd number".format(number))
Validation of user input
Although above code works but there is a caveat. We have to check the input of user. What if user mistakenly enter an invalid number (or a string) then our program will crash with error exception on function int()
. For that we have to surround that part with Try-Except block. We can also compact our output by replacing newline
with tab
when writing each number on screen. Then our source code would be as following:
import sys print("*** Find All Odd Numbers ***") user_input = input("Please enter a number: ") try: max_number = int(user_input) except ValueError: print("Input was not a valid number.") sys.exit(1) if max_number < 0: print("Please enter a positive number.") else: print("Displaying odd numbers from 0 to {0}".format(max_number)) for number in range(max_number): if number % 2 > 0: print("{0}".format(number), end='\t')
You can also find above code in Github’s Gist here.
You may also be interested in following articles: